Modifiers in Unity3D
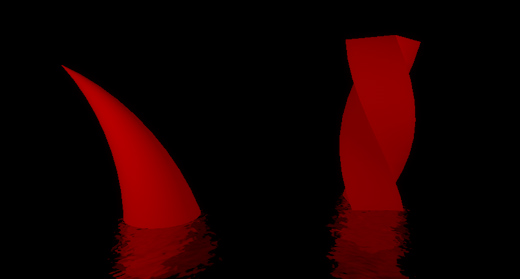
Ringo from FlashBookmarks asked me if there were modifiers similar to AS3Dmod in Unity3d. I searched for something similar some time ago, but didn't find anything interesting.
However, when I was starting C# scripting, I ported two of the modifiers from AS3Dmod – Bend and Twist. So I thought to share them with everyone. Don't expect much, it's not the full library, just two classes. If I have some free time, I'll look into how to implement stacking, since for the moment you cannot apply two modifiers to the same object (the second one won't have any effect).
To play around with them grab this package and import it into Unity. Keep in mind that the modifiers work at runtime, so you won't see any effects in the editor until you run the game. If you want to animate them, just add another script that will have a reference to the modifier instance and change it's properties at each Update() call.
If you are interested in this kind of "bricolage" with 3D geometry, I recommend to take a look at the Procedural Examples project provided by Unity. You will find there some highly interesting samples like dynamic extrudes or perlin noise. It's a great starting point to explore runtime geometry transformation.
Of course, take a look into the sources of the modifiers too! You'll see how simple it is to access the geometry at runtime and do some modifications. Basically it goes like this:
MeshFilter mfilter = GetComponent(typeof(MeshFilter)) as MeshFilter;
mesh = mfilter.mesh;
Vector3[] vs = mesh.vertices;
int vc = vs.Length;
for (int i = 0; i < vc; i++) {
// Modify your vertices here
}
mesh.vertices = vs;
mesh.RecalculateNormals();
Things worth notice: in Unity a vertex is just an instance of Vector3 – there isn't any special class for this, as in Flash-based 3D engines.
You can see a bit of the casting-drama I need to do (lines 1-2) to get to the object holding the actual geometry information (Mesh). That's a bit annoying in C#, with JS that code would be more readable. Anyway, it's better to make that only once, in Start() and not at every Update().
To get correct lightning effects on the materials, do not forget to call mesh.RecalculateNormals() after you've modified the positions of the vertices.
If you want to modify your mesh continuously (i.e. to animate it) it's necessary to keep the original array of vertices, because the code above overwrites the original positions. Take a look at the source code in the package to see how I did it.
That's all for now, hope you enjoy it!